OOPS stands for Object-Oriented Programming Systems. It is a programming paradigm that is based on the concept of "objects", which can contain data and code that manipulates that data. OOPS concepts include:
Encapsulation: the process of hiding the internal details of an object from the outside world.
Inheritance: the ability for one class to inherit the properties and methods of another class.
Polymorphism: the ability for a single function or method to work with multiple types of data.
Abstraction: the ability to focus on the essential features of an object, while ignoring its non-essential details.
Classes and objects: classes are templates for creating objects, which are instances of a class with their own unique properties and methods.
Interfaces: A way to define a contract for a class, that the class must implement.
These concepts work together to help developers create more organized, efficient, and maintainable code.
OOPS concept uses:
Object-oriented programming (OOP) is used in many areas of software development and has a number of advantages, some of the main uses of OOPS are:
Modularity: OOP allows for the creation of self-contained objects that can be easily reused in other parts of the code, making the development process more efficient.
Abstraction: OOP allows for the abstraction of complex systems into manageable objects, making the code more readable and easier to understand.
Inheritance: OOP allows for classes to inherit properties and methods from other classes, reducing the amount of redundant code.
Polymorphism: OOP allows for a single function or method to work with multiple types of objects, making the code more flexible and adaptable.
Encapsulation: OOP allows for the hiding of an object's internal details, making the code more secure and maintainable.
Event-Driven Programming: OOP allows for event-driven programming, which is a way of structuring code around events that occur in the system, such as a button click.
GUI programming: OOP is very useful for graphical user interface (GUI) programming, as it allows for the creation of reusable, modular objects that can be easily manipulated to create a variety of different user interfaces.
Game Development: OOP is widely used in game development, as it allows for the creation of modular and reusable game objects, such as characters, enemies, and items.
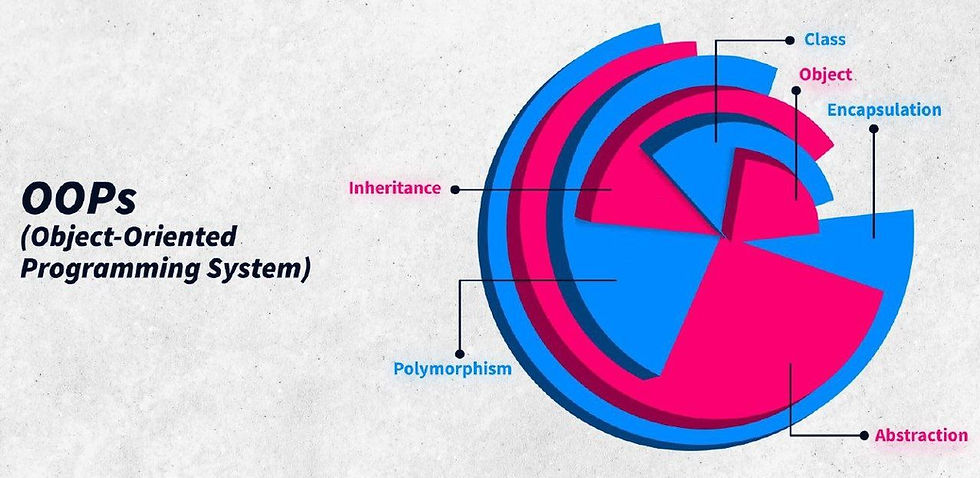
OOPS concept in java
Java is an object-oriented programming (OOP) language, and it supports all the major OOP concepts including:
Encapsulation: Java uses the concept of encapsulation to hide the implementation details of a class from the outside world. This is achieved through the use of private variables and methods, which can only be accessed by other methods within the same class.
Inheritance: Java allows classes to inherit properties and methods from other classes, through the use of the "extends" keyword. This allows for the creation of a parent-child relationship between classes, where a child class can inherit the properties and methods of a parent class.
Polymorphism: Java allows for polymorphism through the use of interfaces and abstract classes. A single method can be written to work with multiple types of objects, which makes the code more flexible and adaptable.
Abstraction: Java provides the ability to create abstract classes and methods, which can be used to define a contract for a class without providing a concrete implementation.
Interfaces: Java uses interfaces to define a contract for a class, that the class must implement. Interfaces are a way to define methods without providing an implementation.
Classes and objects: Java uses classes and objects to implement the OOP concepts. A class is a blueprint for creating objects, and objects are instances of a class with their own unique properties and methods.
Java also support other OOP concepts like: 7. Constructors: which are special methods that are used to initialize the state of an object when it is created.
Overloading: which is the ability to define multiple methods with the same name but with different parameter lists.
Overriding: which is the ability to provide a new implementation for a method that is already provided by a parent class.
OOPS concept in python
Python is an object-oriented programming (OOP) language, and it supports all the major OOP concepts including:
Encapsulation: Python uses the concept of encapsulation to hide the implementation details of an object from the outside world. This is achieved through the use of the "private" and "protected" access modifiers, which are not explicitly defined in the language but are conventionally indicated by a single underscore prefix.
Inheritance: Python allows classes to inherit properties and methods from other classes, through the use of the "class" keyword. This allows for the creation of a parent-child relationship between classes, where a child class can inherit the properties and methods of a parent class.
Polymorphism: Python allows for polymorphism through the use of duck typing, which is the idea that if an object behaves like a certain type, then it is considered to be that type. This means that a single method can be written to work with multiple types of objects, which makes the code more flexible and adaptable.
Abstraction: Python provides the ability to create abstract classes and methods through the use of abstract base classes(ABC) and abstract methods.
Interfaces: Python does not have a built-in concept of interfaces, but it can be simulated through the use of abstract base classes (ABCs) or abstract methods.
Classes and objects: Python uses classes and objects to implement the OOP concepts. A class is a blueprint for creating objects, and objects are instances of a class with their own unique properties and methods.
Constructors: Python uses the special method init() to initialize the state of an object when it is created.
Overloading: Python does not support method overloading, but it can be simulated by using default parameter values.
Overriding: Python allows methods to be overridden in a subclass, by defining a method with the same name as in the parent class.
Comments